In modern web development, the react, angular, and vue frameworks being implemented are the dominant players on the scene. However, Building a simple JavaScript web app without frameworks offers a refreshing alternative.
But these tools usually speed up your developments with a more complex overhead, resulting in overhead costs and the notorious learning curve to master the applications.
Building Simple JavaScript Web Applications Without Frameworks offers a refreshing perspective, showing how you can create dynamic and interactive web apps with just vanilla JavaScript—without the need for any heavy frameworks.
How much simpler is the process without this heavy stuff?
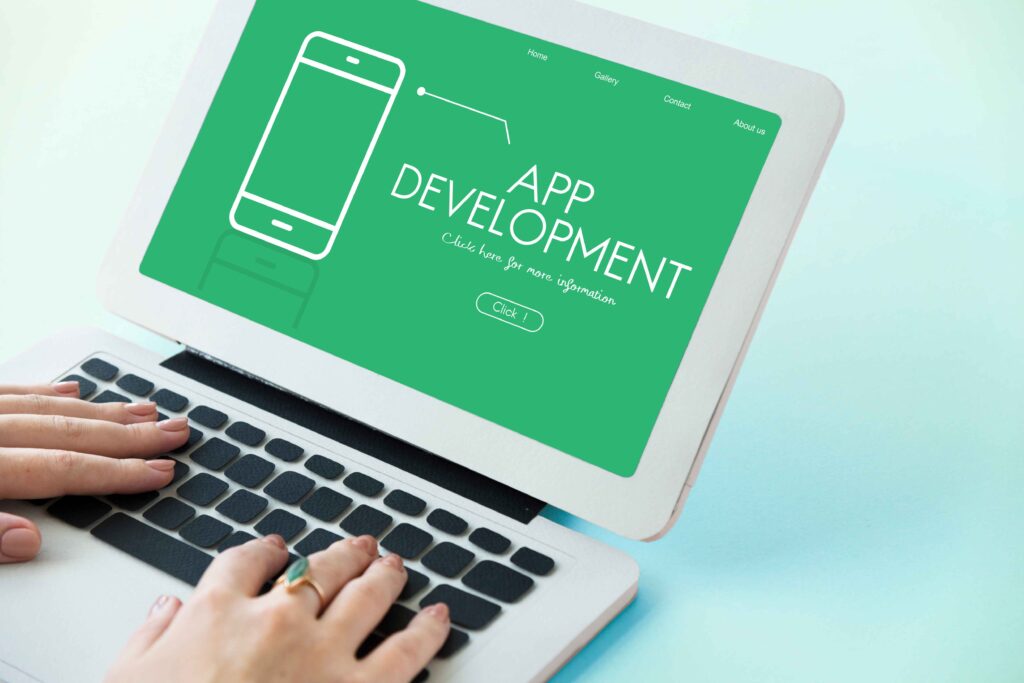
In this post, we will work step by step on how you can create simple javascript web applications using vanilla JavaScript with no frameworks.
We will cover all the essentials such as setting up your app, managing state, handling events, and rendering dynamic content. This will make you better understand how to work with the DOM and manage data flow in a more lightweight, flexible way.
Let’s dive in!
Why Build a JavaScript Web App Without Frameworks?
Before we dive in, let’s talk about why you might want to build an app without a framework
- Lightweight and Fast: Without the overhead of a framework, your app’s size is smaller and can load faster.
- More Control: You have complete control over how the app is structured, without any predefined conventions that frameworks impose.
- Learning Fundamentals: It will make you understand the fundamental web development concepts like HTML, CSS, JavaScript, DOM manipulation, and event handling.
- No Dependencies: It’s less dependent on the external codebase, thereby easier to debug and maintain.
With that in mind, let’s build a simple web app—a task manager app—where you can add, remove, and toggle tasks.
Step 1: Set Up the Project Structure
Let’s start by creating a basic project structure. We’ll need
- An index.html file for the markup.
- A styles.css file for styling.
- A script.js file for JavaScript.
Here’s how our folder structure might look
/task-manager-app
├── index.html
├── styles.css
└── script.js
The HTML (index.html)
We will now define the structure of our app, which will include a header, a form to add tasks, and a list to show them.
Explanation
We have an input field to add tasks and submit them using a button.
Inside an unordered list (<ul>), the tasks will be printed.
We add script.js to handle our JavaScript functionality.
Step 2: Styling the App (styles.css)
We add some basic styling to make our app look relatively good.
Explanation
- We give the app a clean, simple layout with some padding and a centered design.
- Each task will have a background color, and completed tasks will be styled with a line-through effect.
Step 3: Functionality in Action through JavaScript (script.js)
Now let’s bring in some JavaScript to handle the creation of tasks, removal of tasks, and toggling.
1. Adding Event Listeners
We need to add event listeners for submitting the form that adds tasks and to include the marking of tasks as completed.
Explanation
- We add an event listener to the form to handle task submission. When the form is submitted, we create a new list item (<li>) with the task text.
- Each task can be clicked to toggle its completion state by adding/removing the completed tasks.
The tasks are dynamically added to the <ul> in the HTML.
Step 4: Add More Functionality
We can include a delete task feature as well. We’ll add a delete button beside every task.
Explanation
- For each task, we create a delete button (<button>).
- The delete button has an event listener that removes a task from the list when clicked. We use e.stopPropagation() to ensure that the click event does not complete the task when deleting.
Step 5: Final Touches
Your simple task manager app is now complete! With only a few lines of JavaScript, we’ve managed to
- Add tasks dynamically.
- Toggle task completion.
- Remove tasks from the list.
You can enhance this app further by adding features like task persistence (saving tasks to localStorage), prioritizing tasks, or even implementing a due date. But this basic version is a solid foundation.
Conclusion
Building a web application with vanilla JavaScript is an excellent way to learn the basics of web application development, and in the process, you can make something useful.
Of course, frameworks tend to speed up development cycles, but for simple projects, they are often unnecessary. You end up understanding how to push around the DOM, listen to events, and keep track of state on your own.
So the next time you are building some small web application, consider building it framework-free. You will find that surprisingly, vanilla JavaScript can be quite powerful.
Key Takeaways
- Lightweight & Fast: Framework-less web app development leads to quicker loading and smaller app sizes.
- Full Control: Without conventions, you have full control over app structure and design.
- Fundamentals Matter: Framework-less development forces you to learn fundamental web concepts such as DOM manipulation, event handling, and state management.
- No Dependencies: Fewer external libraries mean the app is easier to debug and maintain.
- Simple Web Apps: Frameworkless development is the best for little projects, such as a task manager application, where you have the ability to add, turn on/off, and remove tasks effectively.
- Power of Vanilla JS: Vanilla JavaScript enables you to do complex tasks with less overhead and improved awareness of the lower-level code.
- Frameworks Are Optional: For small, straightforward apps, vanilla JavaScript usually provides an easier and faster solution.