Debouncing is a powerful concept in JavaScript that drastically improves performance in web applications-specially those that handle frequent, event-driven activities.
It starts from search input fields up to those resizing or scrolling events, where debouncing limits the calls of the expensive function by introducing a delay.
This means that the function is performed only after the user has stopped triggering the event for a specified time.
In this post, we will dig deeper into debouncing, practical examples with their usage, and best practices for using this technique.
What is Debouncing?
Debouncing is a practice in programming used to prevent very time-consuming functions, such as handling user input or events, from being called too frequently.
It works by setting a timeout to delay the execution of the function until some idle time has passed.
When the event is triggered again before the timeout has expired, the previous timeout is cleared and a new one is set.
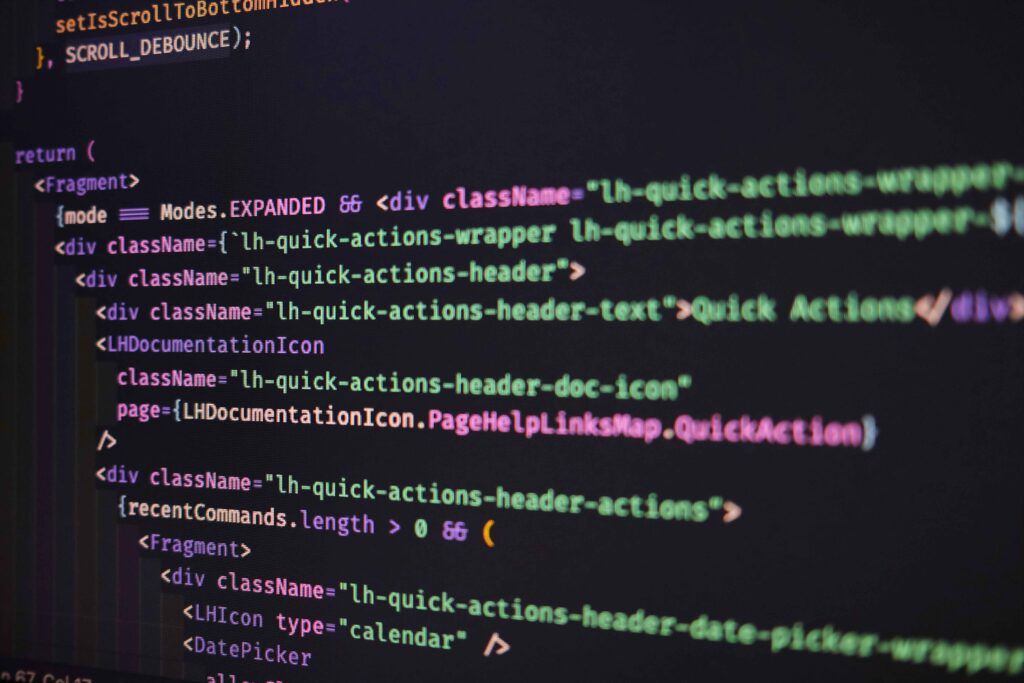
In other words, debouncing ensures that a function is called only once after a series of repeated events, such as typing in a search box or resizing a window.
This is particularly useful for preventing performance bottlenecks in applications.
Debouncing Example
Search Input Field
A user is typing in a search input field and, with each keystroke, an API call is made to fetch search results. Without debouncing, each keystroke may trigger an API request, creating a huge amount of unnecessary requests that degrade performance and user experience.
Debouncing can delay the API call until the user stops typing for a given period.
How Debouncing Works
Practical Example
Let’s create a simple debounce function. Our goal is to delay the call of the function (in this case, an API request) until the user has stopped typing for, say, 300 milliseconds.
The Debounce Function
Here’s a simple debouncing function
Explanation
- func: The function to be debounced.
- delay: The delay (in milliseconds) before the function is executed.
- timeout: A variable to keep track of the timeout ID.
The debounce function returns a function which, if the event is fired again, cancels the previous timeout so that the original function will only run after the user stops firing the event for a specified amount of time.
Example with Search Input
Let’s create an example where an API request is made when the user types something in a search field.
Explanation
The debouncedSearch function is the debounced version of fetchSearchResults. It calls it on every input event.
The query results refresh only when it has spent 300 milliseconds idle, so the actual API request is only when the user has stopped typing.
Best Practices for Debouncing
Set an Appropriate Delay
Choose the right delay. A very short delay would not help improve performance significantly, while too long a delay could make the user experience bad since the application feels unresponsive.
For instance, 300 milliseconds is a good starting point for input events such as search fields, but you might want a longer delay for resize or scroll events.
Use Debouncing for Expensive Operations
The best function for the use of debouncing is functions that incur expensive operations; those are API calls, DOM manipulations, or complex calculations.
Take window resize or scrolling long lists of items-these trigger thousands of events per second, and debouncing those events makes sure your application won’t block.
Do Not Debounce All Events
You should not debounce all events. Simple button clicks or form submissions typically don’t require debouncing since they occur rarely.
Debounce only those events which tend to occur multiple times in rapid succession and, hence, bring performance problems.
Debounce DOM Events, Not UI Interactions
Debouncing works well for events that trigger changes to the DOM or external requests. However, if you debounce interactions like button clicks, it could lead to a confusing user experience.
If a user clicks a button multiple times and debounces the event, the button might seem like it isn’t working.
Combining Debouncing with Throttling
You can use debouncing along with throttling. Throttling ensures the function at a constant interval while debouncing waits for the event to stop.
Throttling limits the operation to occur only at certain speeds, which’s why it usually applies on activities like scrolls but debouncing normally applies when executing expensive operation-like API call scenarios.
Debouncing and JavaScript Framework
If you are working with modern frameworks like React, Vue, or Angular, you can still apply debouncing, although there may be built-in solutions that handle it for you.
For example, in React, you can use hooks like useEffect along with useState to implement debounced input fields.
Many libraries also exist, such as Lodash’s debounce function, which simplifies implementation and provides added flexibility.
Use cases for debouncing
- Limiting the Number of API Calls When a user types a query into the search input fields.
- Window Resize Events: Minimize layout calculations as much as possible.
- Scroll Events: Avoiding Performance Hits While Listening for Scroll Actions.
- Button or Link Clicks Avoid accidental double clicks on important buttons like “Submit”.
conclusion
It’s a pretty simple but quite efficient technique in improving performance on JavaScript. Debouncing reduces expensive function calls by curbing the rate at which they happen, thus guaranteeing a more fluid user experience without straining your system needlessly.
Whether dealing with search inputs, resizing windows, or scroll events, this is how debouncing helps to make your application respond quickly with less load in the browser.
With proper implementation and best practices, debouncing becomes a great addition to your list of performance optimization helpers.
Now at Codeneur, efficient code is where power lies; it’s another part of those tricks we try and implement for user experiences.
Key Takeaways
Debouncing is a technique through which the performance is improved by limiting the frequency of costly function calls, such as API requests or DOM manipulations.
It does this by ensuring that the function being called will not be executed until a defined idle time has passed-thus firing the function only once.
A common one would be that the search input field uses debouncing, where a user typing in it doesn’t cause multiple unfortunate API calls, because it waits for the user to stop typing.
Best practices
- Impose an ideal delay of around 300 ms for search, longer for resize/scroll events.
- Debounce any expensive function call, such as API calls, or any very heavy function.
- Debounce anything that is simple-action-like click of buttons or submission of forms.
- Combining debouncing with throttling: Unlike debouncing, which indicates there is no more activity for a given amount of time, throttling allows you to capitalize on the period of time that surrounds an expensive function.
- Support across frameworks: Modern JavaScript frameworks (React, Vue, and angular) allow debouncing; libraries like Lodash provide built-in support.