Webpack is a great tool for full-stack JavaScript development, bundling both client-side and server-side code efficiently. It further optimize assets like JavaScript, CSS, and images, thereby improving performance and reducing load times.
It makes the integration of the front-end frameworks, such as React, smooth with the back-end technologies, like Node.js. Its major features of code splitting, lazy loading, and tree shaking make apps that are scalable and high-performing.
In this guide, let’s walk through the essentials of setting up Webpack for full-stack development-from the bundling of assets to getting your front-end and back-end code optimized for production readiness.
- What Is Webpack and Why Use It?
- Setting Up Webpack for Full-Stack Development
- Configuring Webpack for Front-End (React/Angular/Vue)
- Configuring Webpack for Server-Side (Node.js/Express)
- Advanced Webpack Features for Full-Stack Development
- Best Practices of Full Stack Webpack Configuration
- Common issues with Full-Stack Webpack Projects
- Conclusion
- Key Takeaways
- FAQ’s
What Is Webpack and Why Use It?
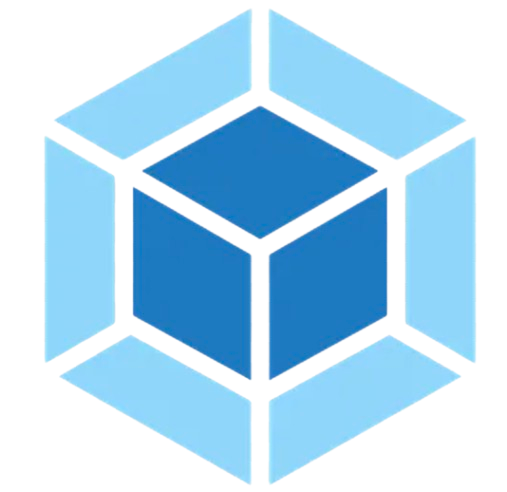
Main Features of Webpack
- Module Bundling: Webpack bundles all your front-end and back-end code, including your JavaScript, CSS, images, and other assets into optimized, smaller files: called “bundles”. Less HTTP requests are needed in order to load an application, which improves performance.
- Code splitting allows splitting your application into pieces. During the start time, only that which is needed will be loaded by Webpack. It’s a fantastic addition to the loading times with supporting non-critical code lazy loading.
Key takeaways
- Loaders and plugins: These are used to load Webpack to preprocess the files like converting from sass to CSS or JSX to JavaScript. They are also used as plugins for improving the build process like minification or adding environment variables.
- Tree Shaking and Optimization: Tree shaking is the removal of unused code from the final bundle. Webpack analyzes your code and deletes dead or unused imports in the process, which leads to a smaller and more optimized bundle.
Common Use Cases in Full-Stack Development
- Optimizing front-end assets with Webpack: Optimization of the kind to be used client-side like JavaScript, CSS and images by minimizing code compressing them in the same sense, as well as newer JavaScript syntax for production purposes.
- Node.js Applications Server-Side Code: Webpack can also bundle the server-side code in Node.js, optimizing it for production, especially for applications that have server-side rendering (SSR), where Webpack can bundle and serve both client and server code seamlessly.
Using all these features, Webpack enhances both the front-end and back-end workflow in full-stack development and makes applications faster and easier to maintain.
Setting Up Webpack for Full-Stack Development
Installing Webpack
To get started with using Webpack for a full-stack project, you first need to install Webpack and its dependencies. This is how you do it:
1. Install Webpack and Webpack CLI
npm install --save-dev webpack webpack-cli
2. Install Babel (for JavaScript transpiling)
To use modern JavaScript features (like JSX or ES6), you need Babel
npm install –save-dev babel-loader @babel/core @babel/preset-env @babel/preset-react
3. Install Webpack Dev Server (for development)
npm install –save-dev webpack-dev-server
4. Install Loaders and Plugins (e.g., CSS, image files)
npm install –save-dev css-loader style-loader file-loader
Once you have the dependencies installed, you would configure Webpack by creating a `webpack.config.js` file in order to explain how your assets should be handled and bundled.
Basic Project Structure
A regular full-stack JavaScript project can look like this
/my-fullstack-app
/client (React front-end)
/src
/components
/assets
index.js
/server (Node.js/Express back-end)
/src
/routes
/controllers
server.js
package.json
webpack.config.js
Webpack is used mainly to bundle the front-end assets (JavaScript, CSS, images) located in the `/client` directory. For the back-end (`/server`), if needed, Webpack may be used to bundle the Node.js code or used for server-side rendering.
Webpack will take care of the development environment and optimize the app for production.
Configuring Webpack for Front-End (React/Angular/Vue)
Webpack for React (Example)
Set up Webpack for a React-based application
Create webpack.config.js: This configuration will decide how Webpack processes and bundles the React code.
// webpack.config.js
const path = require('path');
;
module.exports = {
entry: './src/index.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js',
},
module: {
rules: [
test: /\.jsx?$/,
exclude: /node_modules/,
use: 'babel-loader',
], },
resolve: {
extensions: ['.js', '.jsx'],
},
};
Using Babel for JSX/ES6 Compatibility
Webpack processes the JSX/ES6 source through Babel to compile JSX/ES6 in compatible JavaScript to the browsers. The babel-loader makes.jsx file process all of the Babel Preset needed. For instance @babel/preset-react.
Code Splitting and Lazy Loading: Using dynamic imports to split a large React application into smaller pieces, then apply lazy loading.
const Component = React.lazy(() => import('./Component'));
Handling Styles and Assets
CSS, Sass, and Less: Installation of loaders to handle styles.
npm install –save-dev css-loader style-loader sass-loader node-sass
In webpack.config.js add styles:
{
test: /\.css$/,
use: ['style-loader', 'css-loader', 'sass-loader'],
}
Image and Font Handling: Using file-loader for assets.
npm install --save-dev file-loader
test: /.(png|jpg|gif|woff|woff2)$/
use: 'file-loader',
}
Development Server and Hot Module Replacement (HMR)
Webpack Dev Server: Install and configure to use it for local development:
npm install –save-dev webpack-dev-server
In webpack.config.js turn on HMR
devServer: {
contentBase: './dist',
hot: true,
},
To set up HMR
one needs to use HotModuleReplacementPlugin
const webpack = require('webpack');
plugins: [
new webpack.HotModuleReplacementPlugin(),
],
This setup provides a convenient development experience enabling hot reloading and asset optimisation
Configuring Webpack for Server-Side (Node.js/Express)
Setting Up Webpack for Server-Side Code
For full-stack development, it’s also possible to bundle server-side code with Node.js applications using Webpack. Although client side bundling is unique from that, server side preparation by Webpack will allow you to get your application codes prepared to run in a Node.js context. You configure Webpack in the same manner that you do for the client, but there are several unique modifications to handle for server-side modules.
1. Setting Up Webpack for Node.js
Specify in `webpack.config.js` to include the `target` be set to `node` that tells Webpack to recognize that the output is likely to be served up from a Node.js server of some sort:
module.exports = {
target: 'node',
entry: './src/server.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'server.bundle.js',
},
;
2. Client-Side vs. Server-Side Bundling
Client-side bundling: opt assets for the browser
Server-side bundling: prepares an app to run on Node.js server – no optimizations to the asset for a particular browser
Optimizing Back-End Code using Webpack
- Tree Shaking: Same thing as frontend, removes unused code that’s bundled into server code so that only the actual modules used get included inside the bundle.
- Production Mode: Run Webpack in production mode to optimize server-side code. This minimizes the code, improves performance, and enables advanced optimizations such as minification and dead code elimination.
Using Express for Server-Side Rendering (SSR)
For server-side rendering (SSR) with React (or other frameworks), Webpack bundles both front-end and back-end code, and the server can render the initial HTML. Configure Express to serve the bundled code
app.get('*', (req, res) => {
res.sendFile(path.resolve(__dirname, 'dist', 'index.html'));
});
Webpack can even handle full-stack apps in terms of managing server-side assets, like API routes and static files, so all components are bundled very efficiently.
Advanced Webpack Features for Full-Stack Development
Code Splitting
Code splitting is a method to boost performance, since it allows you to divide your application into smaller and easier-to-handle pieces. When using Webpack, you can automatically and manually split code so that only parts which are in use by your application are loaded during runtime.
Dynamic Imports Load some parts of your application on demand
const Component = React.lazy(() => import('./Component'));
Lazy Loading: This technique loads parts of the application that are not required to be loaded on the first run, thereby shortening the time it takes for the first load. Webpack splits the code into smaller chunks that load on demand.
Tree Shaking
Tree Shaking is a technique removing unused code from the final bundle. This is the optimization with the highest impact, reducing the size of production builds. For tree shaking, you have to use ES6 modules (`import`/`export`) that can be evaluated by Webpack for dead code elimination.
Tree Shaking configuration: Set the webpack.config.js to production mode
mode: ‘production’,
ES6 Module Support: Tree shaking will work with ES6 imports and exports. Ensure your codebase is ready using `import` and `export` statements instead of CommonJS (`require`/`module.exports`).
Minification and Optimization
1. JavaScript Minification: Use Terser (or UglifyJS) for JavaScript minification:
npm install –save-dev terser-webpack-plugin
2. CSS Minification: Use PostCSS with plugins like cssnano to minify and optimize CSS files:
npm install –save-dev postcss-loader cssnano
Environment-Specific Configuration
In Webpack, you may have configuration values that are development or production specific. This can be useful because there usually is a value which should use toggle switches.
Those might include source map in debug only and minification
mode: process.env.NODE_ENV === ‘production’? ‘production’ : ‘development’
Best Practices of Full Stack Webpack Configuration
Modularizing Your Webpack Configuration
Modularizing your Webpack configuration is very much important to make it clean and scalable. What you would do is given below:
webpack-merge: You will let you separate development and production configurations but keep common configurations in common settings.
Now, you would maintain an uncluttered webpack.config.js that will further help you to manage one easily.
npm install –save-dev webpack-merge
Example:
const merge = require('webpack-merge');
const commonConfig = require('./webpack.common.js');
const prodConfig = require('./webpack.prod.js');
module.exports = merge(commonConfig, prodConfig);
Break Configurations for Readability: Break your configuration up into files for common, development, and production. Moreover, You can then very easily concentrate on specific configurations without messing up a single file.
Version Control for Webpack Assets
It avoids issues with caching, and users get the assets that have been freshly loaded.
Use content-based hashing in your output filenames:
output: {
filename: '[name].[contenthash].js',
},
Files that are not modified retain their hash, and modified files obtain a new hash and invalidates the cache.
Monitoring Bundle Size
Bundle size can be monitored by using packages such as Webpack Bundle Analyzer.
npm install --save-dev webpack-bundle-analyzer
Visualizing your bundle allows you to catch large dependencies that you then can optimize to improve load times and performance.
Deployment of Full-Stack Applications Using Webpack
Building Production-Ready Code
To make your application production-ready, run Webpack with the `–mode production` flag:
webpack –mode production
This allows optimizations such as minification, dead code elimination tree shaking), and more, for assets. Webpack will, out of the box, minify your JavaScript and optimize CSS so the build is smaller and faster.
Lastly, ensure assets like images are optimized, using `image-webpack-loader` that compresses, which improves performance again. Output filenames should have a content hash for cache busting:
output: {
filename: '[name].[contenthash].js',
},
Integration with Deployment Pipelines
Webpack Integration with CI/CD workflows will make sure that your build process is automated and consistent across environments.
GitHub Actions and Jenkins can be used to trigger a Webpack build when code is pushed to a repository.
1. GitHub Actions: Configure a CI pipeline to run `webpack –mode production` every time code is pushed
- name: Build and deploy
run: |
npm install
npm run build
Common Deployment Scenarios
1. Heroku: Deploy a full-stack app by setting up a Node.js server with Webpack’s production build.
2. AWS/DigitalOcean: Use something like Elastic Beanstalk from AWS or a Docker container from DigitalOcean to host your front-end and back-end code. Ensure that your server delivers the static Webpack assets from the `/dist` folder.
Webpack will then ensure your production-ready code is optimized for super-fast loads and efficient performance on any platform.
Common issues with Full-Stack Webpack Projects
1. Module resolution errors : Sometimes when a module is not available in your code, the result would be a ‘Module not found’ error.
Thus, ensure all the paths are correct, and you have no spelling mistake on your imports. The following code in webpack.config.js helps solve such errors, define a few directories in which Webpack shall look:
resolve: {"}
extensions: ['.js', '.jsx', '.json'],
}
2. Loader/Plugin Errors: Issues with loaders or plugins (e.g., Babel or CSS loader) often stem from misconfigurations. Double-check your loader rules and ensure you’ve installed the necessary dependencies, such as `babel-loader` or `style-loader`.
3. Performance Issues in Large Applications: Large applications take much longer to build. Split your code using Webpack’s code splitting feature to make the initial bundle size smaller, and apply tree shaking to eliminate unused code.
Performance Tips
1. Lazy Loading and Caching: Use lazy loading to load non-essential code later and apply Web pack’s `dynamic import` for components that need to be loaded only when necessary:
const Component = React.lazy(() => import('./Component'));
2. Webpack Caching: You can use the built-in Webpack caching mechanism through contenthash in filenames. In development, you may want faster incremental builds: use the cache-loader or hard-source-webpack-plugin.
All these approaches will help address common pain points and tune Webpack builds to speed up both your performance and your build time for full-stack projects.
Conclusion
In summary, adding Webpack to your full-stack development workflow is highly efficient and scalable.
Therefore, using Webpack to automate tasks such as bundling, code splitting, and optimization ensures to make the process much easier while it develops your projects for it to be fast and powerful for both the front-end and the back-end.
This application-building tool will improve your life by integrating modern JavaScript frameworks, handling complex assets, and speeding up the loading of web pages.
Dig deeper into Webpack and learn full-stack development with Codeneur, your go-to Bootcamp for comprehensive live classes and expert guidance.
Key Takeaways
- Module Bundling: Webpack optimizes client-side as well as server-side code through bundling the assets such as JavaScript, CSS, and images into smaller ones.
- Code Splitting: Loads only required components of the app to enhance the performance.
- Tree Shaking: Removes unused code to minimize bundle size.
- Loaders & Plugins: Assist in preprocessing files as well as to improve the building process.
- Optimizing Server-Side: Webpack also bundles Node.js code for deployment, including the SSR configurations.
- Production Readiness: Webpack optimizes assets for more efficient loading and improved performance.
- Integration: Can easily integrate into CI/CD processes for repeatable builds