Arrays are one of the most important data structures in JavaScript. You will find yourself constantly dealing with arrays when handling user data, manipulating UI elements, or even conducting calculations.
There is, however, an overwhelming number of array methods provided in JavaScript such as.map(),.filter(), and.reduce() if you are just starting out with them.
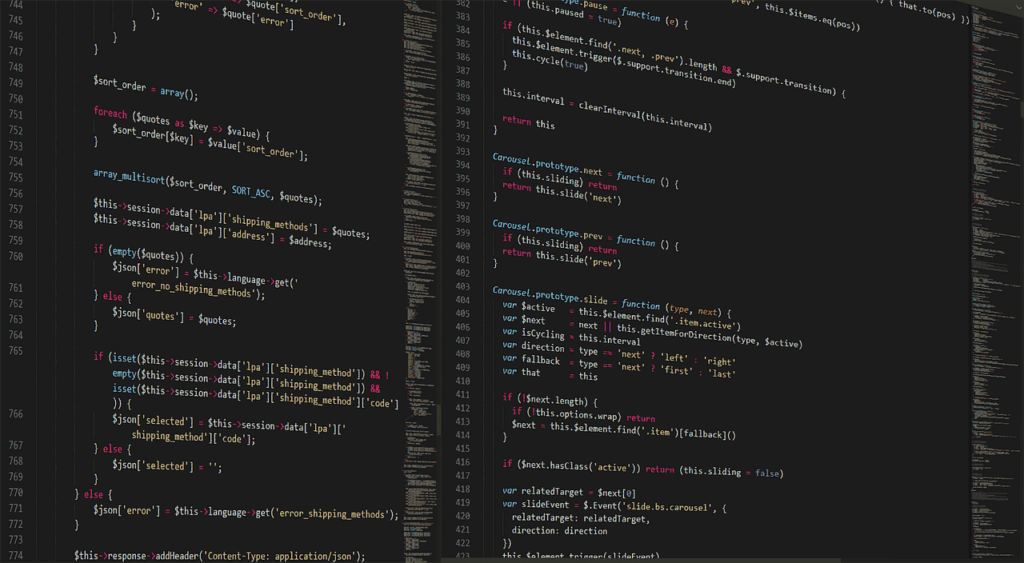
Let’s dive in on some very practical examples here with clear explanations and some hidden gems to really get the best out of them.
What are Array Methods in JavaScript?
Arrays in JavaScript are supplied with built-in methods for you to manipulate, transform, and filter the contents of arrays. These can often make your code more concise, readable, and efficient.
The most used of these methods include
Each one of them is doing a single task; when and how you use it can make a huge difference between writing clean and effective code.
Understanding .map()
The .map() lets you transform the elements of an array by applying a function to each element. It returns a new array with all its elements transformed, and in no way changes the original array.
Syntax
const newArray = array.map(callback(element, index, array));
In the example below, we have an array of numbers and want to square every number.
In the above code snippet,.map() goes through each number of the numbers array, squares it, and then returns a new array of its squared values.
You can use.map() to both transform data and flatten nested arrays in one line of code
This example maps each inner array to a string, thus flattening this structure
Understanding .filter()
The.filter() method creates a new array with all elements that pass the test implemented by the provided function. It does not change the original array.
Syntax
const filteredArray = array.filter(callback(element, index, array));
Practical Example
If you have an array of numbers and you want to filter out all the even numbers
Here,.filter() returns a new array with only the odd numbers.
Lesser known Trick
You can use.filter() on complicated objects. Suppose you have an array of objects representing products and you need to filter in-stock products
Understanding .reduce()
The .reduce() method is used to accumulate or reduce the elements of an array into a single value. So, it’s extremely powerful and can be used for tasks like summing numbers, flattening arrays, and even more complex operations like grouping data.
Syntax
const result = array.reduce(callback(accumulator, currentValue, index, array), initialValue);
Practical Example
Imagine you have an array of numbers, and you want to find the sum of all the elements
In this example, .reduce() is using its iterator to walk through the array, building up the sum of the numbers.
Obscure Bonus
You can use .reduce() for other aggregation operations. For instance, what if you have an array of people objects and you want to group them by age
This example uses.reduce() to group the people by their age, returning an object where each key is an age and the value is an array of people of that age.
Combining .map(), .filter(), and .reduce()
One of the strong techniques is using these methods in combination to perform more complex tasks. For example, you can use.filter() to select only certain items, then.map() to transform them, and finally.reduce() to aggregate the results.
Example
Suppose you have an array of products and you want to calculate the total price of all available products:
In this example, .filter() excludes out-of-stock items, .map() extracts their prices, and .reduce() adds the prices together.
.forEach() and .find()
Not as strong as.map(),.filter() or.reduce() but in the world of array manipulation, there is also room for.forEach() and.find().
.forEach() function executes a given function on every element in the array but doesn’t return anything.
.find() comes in handy when you have to find the first element for which the function returns true but once it does you can break out of that loop.
Here is an example.
Additional Techniques and Considerations
- Immutable operations: Methods such as.map(),.filter(), and.reduce() leave the original array untouched, making this a preferred property in much modern JavaScript application programming, specifically when using it in functional-style coding.
- Performance considerations: Improvement in readability notwithstanding, take performance into account when working with large datasets. For intensive operations, native loops might be faster.
- Chaining methods: These methods can be chained together to produce elegant, concise solutions to complex problems.
Conclusion
Mastery of JavaScript array methods doesn’t just make code cleaner and easier to read but also opens up the potential for performance improvements. From handling user data manipulation up to the transformation of elements and heavy operations, these methods can, therefore, be used to get things done.
Armed with the knowledge of `.map()`, `.filter()`, `.reduce()`, and other powerful techniques, you’ll be ready to handle every type of work involving arrays with more confidence and higher efficiency.
Our Ultimate Full Stack developers course enables you to utilize these techniques to write elegant solutions, minimize errors, and boost performance.