JavaScript has changed vastly over the years, and with ES6 (ECMAScript 2015) came an array of new features. The spread operator (.), for one, is one such feature that has found universal usage by developers while working with arrays, objects, and even function arguments.
In this blog, we will explore the different applications of the spread operator and how it can make your JavaScript code simpler
What is the Spread Operator?
The spread operator is a syntax which enables you to expand or “spread” array or object elements into another array or object.
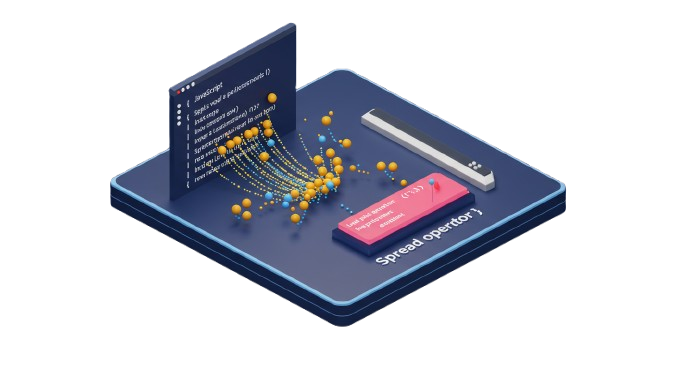
The spread operator employs three dots (.), and its major benefit is that it makes code shorter and readable.
Syntax:
.iterable
Using the Spread Operator with Arrays
The spread operator excels with arrays. Below are some popular use cases:
Copying Arrays: The spread operator can be applied to make a shallow copy of an array.
const arr1 = [1, 2, 3];
const arr2 = [.arr1];
console.log(arr2); // Output: [1, 2, 3]
Merging Arrays: You can merge two or more arrays with the spread operator:
const arr1 = [1, 2];
const arr2 = [3, 4];
const arr3 = [.arr1,.arr2];
console.log(arr3); // Output: [1, 2, 3, 4]
Appending Elements to Arrays: You may add elements into an array using the spread operator along with new elements
const arr = [1, 2, 3];
const newArr = [0,.arr, 4];
console.log(newArr); // Output: [0, 1, 2, 3, 4]
Using the Spread Operator with Objects
The spread operator is also helpful with objects. It makes easy to copy, merge, and update properties.
Copying Objects: It is possible to use the spread operator to copy an object
const obj1 = {a: 1, b: 2};
const obj2 = {.obj1};
console.log(obj2); // Output: {a: 1, b: 2}
Merging Objects: It is possible to merge more than one object into a single object
const obj1 = {a: 1};
const obj2 = {b: 2};
const merged = {.obj1, .obj2};
console.log(merged); // Output: {a: 1, b: 2}
Updating Properties in Objects: Update or add new properties to an object with ease
const obj = {a: 1, b: 2};
const updatedObj = {.obj, b: 3, c: 4};
console.log(updatedObj); // Output: {a: 1, b: 3, c: 4}
The Spread Operator in Function Arguments
The spread operator is really handy when you need to pass elements of an array as individual arguments to a function. For instance
const numbers = [1, 2, 3];
console.log(Math.max(.numbers)); // Output: 3
Shallow vs Deep Copying with Spread
Although the spread operator makes a shallow copy (both of arrays and objects), it can cause strange results when the copied structure has nested objects or arrays.
The spread operator will only replicate references to the nested objects and not the objects themselves.
const arr = [{'a': 1}, {'b': 2}];
const arrCopy = [.arr];
arrCopy[0].a = 99;
console.log(arr); // Output: [{a: 99}, {b: 2}]
Limitations of the Spread Operator
Though it has numerous benefits, the spread operator is not appropriate for deep copying arrays/nested objects.
For deep cloning, there are other methods such as JSON.parse(JSON.stringify()).
Best Practices and Tips
- Use the spread operator whenever you want to clone or merge objects/arrays to enhance code readability.
- Do not use it to clone nested structures deeply unless you know its constraints.
- Have performance in mind, because distributing huge objects or arrays will have some influence in the realm of memory usage and processing time.
Conclusion
The Spread Operator (`.`) is a robust JavaScript feature that renders code cleaner and more efficient by making operations such as copying, merging, and array and object manipulation easier. It ensures immutability, avoiding direct modification of data structures, and forms shallow copies for easier handling.
Knowing when and how to utilize the Spread Operator enables you to write cleaner, more efficient JavaScript code, avoiding redundancy and making code more readable. It is particularly helpful in functions, combining arrays, and modifying objects.
For those aiming to become an expert in JavaScript and web development, Codeneur Bootcamp has extensive courses and practical exercises to take your coding skills to the next level.
Key Takeaways
- The Spread Operator (`.`) is an ES6 feature in JavaScript.
- It allows expansion of elements in arrays, objects, and strings.
- It helps merge arrays or copy objects easily.
- The Spread Operator maintains immutability by avoiding direct mutation.
- It provides shallow copies, i.e., nested items are referenced.
- It can be used for managing function arguments and object modifications.
- The operator enhances code legibility and minimizes redundancy.
- It is compatible with recent browsers but older browsers might need a transpiler.
- It’s critical in contemporary JavaScript development.
- Its application in nested structures should be understood for better effectiveness.