With several features developed over the years to increase developer productivity in JavaScript, one of the most noteworthy is the arrow function. Understanding arrow functions in JavaScript becomes important for developing cleaner, more concise code-everything to do with callbacks, array methods, and event-handling.
Arrow functions have simplified this syntax tremendously by reducing the amount of boilerplate code and further helping one to see this keyword more intuitively.
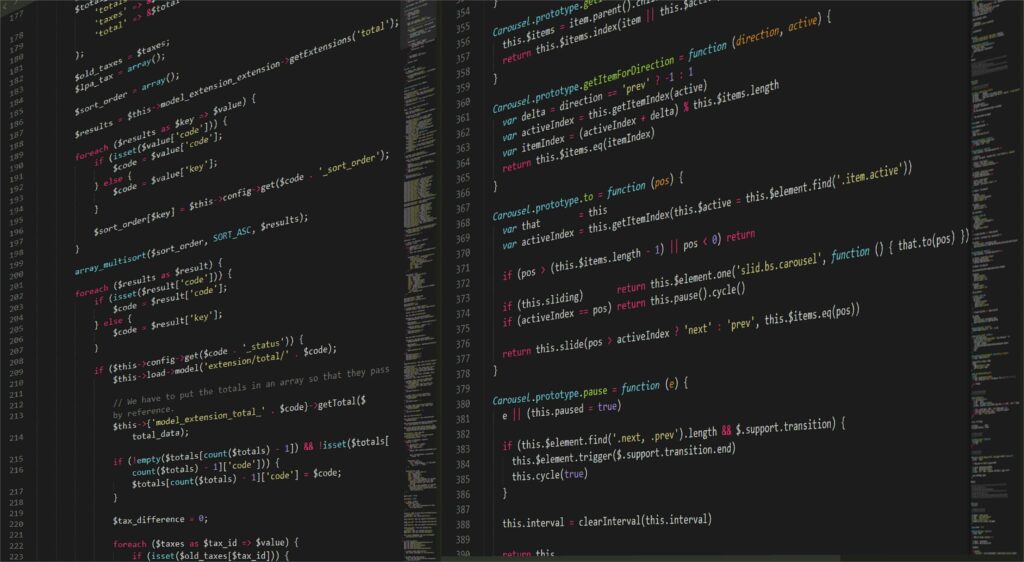
In this article, we will look into the syntax and benefits and the right scenarios of use to take an already dedicated JavaScript programmer to the next level.
What Are Arrow Functions?
Arrow functions in JavaScript allow you to write a function in a much shorter form. They reduce boilerplate code, thus enabling you to define functions with less noise, thus easier to read.
Arrow functions are expressions that act as a replacement for classic or regular functions; they are often used in callback functions when working with array functions such as map(), filter(), reduce(), or simply any functional programming.
The basic arrow function looks like this
It’s that easy! But let’s take a step back and explore what makes arrow functions different from regular function expressions.
The Syntax
Arrow functions have three important components
- Parameters : The parameters in the function follow the same usage as any traditional function, just with the same brackets around parameters. When only one parameter, you don’t need the parenthesis.
- Arrow (=>): This symbol separates the parameters from the body of the function and is the defining feature of arrow functions.
- Body: The body of the function may be either one expression or many statements. When there is only one expression, the results are shown. In the case of several expressions, use curly braces {} and have to include an explicit return statement as well.
Examples
No Parameters
Multi-statements (curly braces required, and explicit return)
Key Features of Arrow Functions
1. Short syntax
Arrow functions are all about minimizing verbosity. You can write your functions in a single line without having to declare function, using curly braces, or return keyword when it comes to simple cases.
Here are the examples of traditional functions and arrow functions by way of comparison
Function using the arrow function syntax
Notice how the arrow function itself removes the need for the return keyword, thus making the code more concise and readable .
2. Implicit Return
If an arrow function is just one expression, it will automatically return whatever that expression outputs. In other words, it is termed an implicit return. So, there’s no need for the explicit declaration of return.
Example
const double = num => num * 2; // Implicit return
The number of statements isn’t relevant with functions. Therefore, you use curly braces along with the return keyword.
3. Lexical this Binding
One of the biggest advantages of arrow functions is how they handle this. In traditional functions, the function dynamically binds this
based on how it is called. Arrow functions, however, do not have their own this.
Instead, they inherit this
from the surrounding lexical scope, which defines the context in which they were created. This is particularly useful in event handlers or when working with callback functions.
Consider this example with a plain function
In the code above, the regular function used within setInterval() has no access to this keyword from the Counter instance. It instead references the global object or undefined in strict mode.
Now, using an arrow function
Now, in the above example, the arrow function inside setInterval() correctly maintains its context to point to the instance of Counter because that is exactly what we are looking for.
When Should You Use Arrow Functions?
Arrow functions are best used in cases where you require concise syntax and lexical this binding. Here are some examples where arrow functions stand out:
- Short Callbacks: The arrow function is mostly a callback. Methods such as map(), filter(), and reduce() frequently use an arrow function.
- Event handlers: Arrow functions can maintain the surrounding context of
this
in event handlers.
- Functional Programming: They come in handy where you are applying higher-order functions, which involve passing functions around. These functions are map(), filter(), and reduce().
- Simple function: With a function consisting of just one expression, the arrow function’s compact syntax makes it more readable than the equivalent function declaration.
Things to Keep in Mind
While arrow functions bring many benefits, there are some limitations to note
- No this Binding: Arrow functions do not bind their own this, so they cannot be used as constructors. You cannot use new with arrow functions.
- No arguments Object: Arrow functions do not have their own arguments object. If you need access to the arguments, you should use a regular function.
- Not Suitable for Methods: If you are defining methods inside an object or class and you want to use this, then a regular function is preferred. Using arrow functions for methods may cause problems because this will not work as expected.
Conclusion
Arrow functions are an important feature of JavaScript. They make code look more readable and easier, particularly when there is a lot of short function use and callback applications.
With this lexical binding for this, it is used excellently in several applications like event handling and functional programming, though one has to be well-informed of the limitations.
The right kind of function for a particular task needs to be selected.
Codeneur is glad to assist you on your way, simplifying complex concepts for you to write great code!
Happy coding!
Key Takeaways
- Concise Syntax: Arrow functions simplify code with shorter syntax, making it more readable.
- Implicit Return: Single-expression functions automatically return the result.
- Lexical this Binding: Arrow functions inherit this from the surrounding context, useful for event handlers.
- Best for Short Callbacks: Ideal for methods like map(), filter(), and reduce().
- Not for Object Methods: Avoid using them as object methods since they don’t bind their own this.
- No arguments Object: Use regular functions if access to arguments is needed.
- Not Constructors: Arrow functions can’t be used with new.